Information about the import API method.
NOTE: This is an Enterprise Feature, it is only available for users who have purchased our Complete Solution Plan.
editFeature
Purpose
Edit a feature in a dataset by passing key-val pairs for attributes and a layerMetaId. If one of the address fields is modified a re-geocode will take place on the server and cause the feature to be moved on the map.
Request URL
PUT https://maps.espatial.com/esWebAPI/rest/v1b/import/{layer_meta_id}/feature
PUT https://maps.espatial.com/esWebAPI/rest/v1b/import/{layer_meta_id}/feature?criteria={"$type":"Equal","attr":"COMPANY","value":"Mills Insurance"}
Request Parameters

Response
[{
"$type": "DefaultLayerMeta",
"created": 1433511718531,
"defaultStyler": {
"$type": "SingleStyler",
"style": {
"$type": "SymbolStyle",
"color": 4292686388,
"size": 30,
"symbolTypeId": 8
}
},
"description": "sample",
"featureSourceId": 5062,
"geoLocationMeta": {
"$type": "AddressGeoLocationMeta",
"attrNames": [
"ADDRESS",
"CITY",
"COUNTY",
"STATE",
"ZIP"
],
"countryCode": "US",
"region": "United States"
},
"geomExportable": false,
"geomType": "POINT",
"id": 4062,
"labelAttr": "COMPANY",
"lastModified": 1433511718531,
"maxResolution": 0,
"minResolution": 0,
"sourceTenantId": 224310,
"title": "sample"
}]
createFeature
Purpose
Create a feature in a dataset by passing key-val pairs for attributes and a layerMetaId.
Request URL
POST https://maps.espatial.com/esWebAPI/rest/v1b/import/{layer_meta_id}/feature
Request Parameters

Response
[
{
"$type": "DefaultLayerMeta",
"created": 1433511718531,
"defaultStyler": {
"$type": "SingleStyler",
"style": {
"$type": "SymbolStyle",
"color": 4292686388,
"size": 30,
"symbolTypeId": 8
}
},
"description": "sample",
"featureSourceId": 5062,
"geoLocationMeta": {
"$type": "AddressGeoLocationMeta",
"attrNames": [
"ADDRESS",
"CITY",
"COUNTY",
"STATE",
"ZIP"
],
"countryCode": "US",
"region": "United States"
},
"geomExportable": false,
"geomType": "POINT",
"id": 4062,
"labelAttr": "COMPANY",
"lastModified": 1433511718531,
"maxResolution": 0,
"minResolution": 0,
"sourceTenantId": 224310,
"title":
"sample"
}]
importURL
Purpose
Perform a bulk update of a dataset by passing the URL of a file.
Request URL
POST https://maps.espatial.com/esWebAPI/rest/v1b/import/url
POST https://maps.espatial.com/esWebAPI/rest/v1b/import/url?url=https://www.espatial.com/sample/sample-short-modified.csv&layerMetaId=108179&overwrite=true
Request Parameters
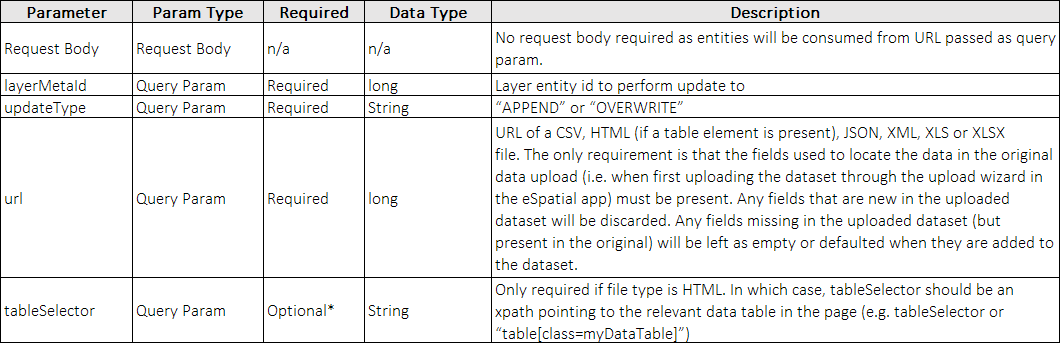
Response
Response body is the number of records/features in the URL that have been counted for processing. Response code is 202/Accepted as the geocoding process can be time consuming.
10
A response header “Column-Match-Summary” is also returned which will give an indication of which fields passed up were processed.
importFile
Purpose
Perform a bulk update of a dataset by POSTing a file.
Request URL
POST https://maps.espatial.com/esWebAPI/rest/v1b/import
Request Parameters

Response
Response body is the number of records/features in the URL that have been counted for processing. Response code is 202/Accepted as the geocoding process can be time consuming.
10
A response header “Column-Match-Summary” is also returned which will give an indication of which fields passed up were processed.
Code Samples
Below are two code samples posting a multipart file to eSpatial for import.
Using C# with RestEasy lib:
static void doMultiPartPostToESpatial()
{
var client = new RestClient("https://maps.espatial.com/esWebAPI/rest/v1b");
client.Authenticator = new HttpBasicAuthenticator("kflood@espatial.com", "***");
var request = new RestRequest("import?layerMetaId=179995&updateType=OVERWRITE", Method.POST);
request.AddHeader("Content-Type", "multipart/form-data");
request.AddFile("file", "c:/inbox/sample.csv", "application/octet-stream");
// easy async support
client.ExecuteAsync(request, response => {
Console.WriteLine(response.Content);
});
}
Using Java with Jersey lib:
private Response doMultiPartPostToESpatial(){
String url = "https://maps.espatial.com/esWebAPI/rest/v1b/import";
final ClientConfig clientConfig = new ClientConfig();
HttpAuthenticationFeature feature = HttpAuthenticationFeature.basic(auth.getEmail(), auth.getPassword());
clientConfig.register(feature);
client = ClientBuilder.newClient(clientConfig);
client.register(MultiPartFeature.class);
// MediaType of the body part will be derived from the file.
FileDataBodyPart filePart = new FileDataBodyPart("file",
new File(filepath),
MediaType.APPLICATION_OCTET_STREAM_TYPE);
FormDataMultiPart multipart = (FormDataMultiPart)new FormDataMultiPart().bodyPart(filePart);
Response res = client.target(url)
.queryParam("layerMetaId", layerMetaId)
.queryParam("updateType", "overwrite")
.request(MediaType.APPLICATION_JSON_TYPE)
.post(Entity.entity(multipart, multipart.getMediaType()));
return res;
}